Exploring time-tests-and-true-JavaScript-objects-puzzles is definitely one of the strongest ones. Which each JS developer MUST know. The key is the ability to check by an individual key arrangement within an object. Welcome to the JavaScript Check If Key Exists Review Guide. Your faithful helper when obtaining object knowledge.
Overview
Feature | Description |
Tool Name | JavaScript Check If Key Exists |
Purpose | Verifying the presence of a key in a JavaScript object |
Usefulness | Crucial for dynamic data manipulation and error handling |
Supported Syntax | ‘key’ in obj, obj.hasOwnProperty(‘key’), obj?.[‘key’] |
Compatibility | Cross-browser, works in all modern JavaScript environments |
Difficulty Level | Beginner to Intermediate |
JavaScript Check If Key Exists JSON
In this regard, let’s slow down and pay homage to the genesis of the JSON data format – JavaScript objects – which play the role of the fingers that interlock to form the beautiful foundation of the JSON data format. As these data structures are versatile, they can be used to store and manipulate information in a structured and organized manner, hence making them an inseparable part of the web development world.
What are Objects in JavaScript?
In JavaScript, objects are named groups of pairs of key-value, wherein the keys act as unique identifiers, and values can be of any type of data, such as other objects, arrays, even functions. These flexible data structures form the integral basis of many applications that have JavaScript programming languages, whether simple or complex.
What are the Keys in JavaScript?
The keys in a JavaScript object are the unique elements that you can utilize to manipulate the defined values. In this case, a key can take the form of a string or even a number allowing for greater flexibility and creativity in how information is packaged and structured into your data.
How to Check if the Key Exists in an Object?
Now, let’s dive into the crux of the matter: In JavaScript Check If Key Exists, suppose that we have an object that is defined as follows: {key1: value1, key2: value2, key3: value3} Now, how can we know whether a specific key is present or not? Certainly, JavaScript has a wide range of methods that allow it to achieve this. Because Every method has its own features and particular applications.
Using the ‘in’ Operator
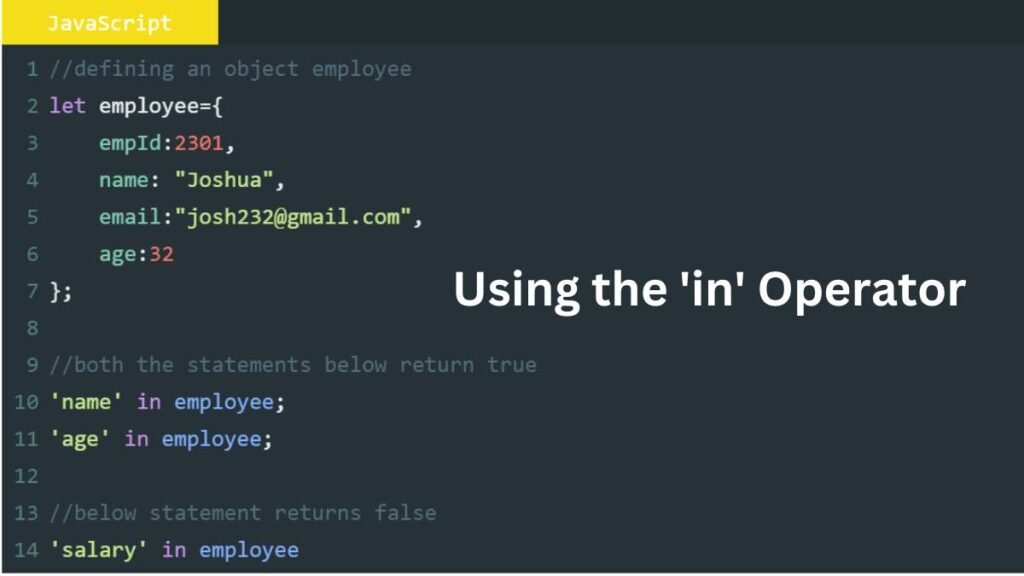
There is the simplest way to check if an object has a specific `‘in‘` key operator. The usage simplicity is accompanied by the elegant syntax that allows you to understand the key violation in a moment, so it has become very popular.
“`javascript
const myObject = { name: ‘John Doe’, age: 30, occupation: ‘Software Engineer’ };
console.log(‘name’ in myObject); // Output: true
console.log(’email’ in myObject); // Output: false“`
Using the Inbuilt ‘hasOwnProperty() Method
Another effective method for checking if a key exist in an object is built-in `hasOwnProperty()` method. This technique proves to be the most effective one when you have to separate the keys that are directly own by the object from those that are having the prototype as their source.
“`javascript
const myObject = { name: As part of my interview process, I have collected some preliminary information about ‘John Doe’ (name, age, and occupation: ‘Software Engineer’).
console.log(myObject.hasOwnProperty(‘name’)); // Output: true
console.log(myObject.hasOwnProperty(’email’)); // Output: false“`
Optional Chaining
The recent releases of JavaScript by introducing optional chaining have made handling of the possibility of keys being undefined or null very convenient. Therefore, checking the use of keys is an easy process now. This advantage can help you eradicate undue mistakes and reduce the mechanical trappings of your code.
Crucial Tips to Remember
In order to make sure that `hasOwnProperty()` is used only when it is necessary to do a specific check for object ownership remember to use this method specifically when you need to strictly check for object keys that are directly owned by the object.
The `’in’` operator can be quite useful for rapid checking of certain keys. Ensure that the approach you use doesn’t suffer from problems related to inherited properties.
Quite often, optional chaining would actually come to your rescue along the way, when being face with uncertain and null values, meanwhile keeping in mind to use it with some amount of discretion to preserve code readability.
Conclusion
Knowing how to see if a key is present in a JavaScript object is like having one more tool in your toolkit; something that every developer should master. No matter whether you’re managing the stack of dynamic data structures, building error-tolerant programmes or just using object-oriented concepts, the things that you have learned will definitely be useful.
So, the next time you find yourself in a JavaScript object exploration conundrum, remember to reach for your trusty “JavaScript Check If Key Exists” toolkit, and let the magic of object manipulation unfold before your eyes.
FAQS About JavaScript Check If Key Exists JSON
Ans. To check if a key is available in a JavaScript object, you can use the `in` operator or the `hasOwnProperty()` method.
Ans. To check if a key exists in a JavaScript Set, you can use the `has()` method.
Ans. To check if a key (index) exists in a JavaScript array, you can use the `hasOwnProperty()` method or the `in` operator.
Ans. To check if a key already exists in a JavaScript object, you can use the `in` operator or the `hasOwnProperty()` method.
Ans. To check if a key exists in a JavaScript object (which can represent a JSON object), you can use the same techniques as checking for a key in a regular JavaScript object – the `in` operator or the `hasOwnProperty()` method.